基本的な演算子
日本語を消す 英語を消す下記URLから引用し、日本語訳をつけてみました。
https://docs.swift.org/swift-book/documentation/the-swift-programming-language/basicoperators
Perform operations like assignment, arithmetic, and comparison.
代入、算術、比較などの演算を実行します。
An operator is a special symbol or phrase that you use to check, change, or combine values. For example, the addition operator (+
) adds two numbers, as in let i = 1 + 2
, and the logical AND operator (&&
) combines two Boolean values, as in if enteredDoorCode && passedRetinaScan
.
演算子は、値を確認、変更、または結合するために使用する特別な記号または語句です。 たとえば、加算演算子 (+
) は、let i = 1 + 2
のように 2 つの数値を加算し、論理 AND 演算子 (&&
) は、if enteredDoorCode && PassedRetinaScan
のように 2 つのブール値を結合します。
Swift supports the operators you may already know from languages like C, and improves several capabilities to eliminate common coding errors. The assignment operator (=
) doesn’t return a value, to prevent it from being mistakenly used when the equal to operator (==
) is intended. Arithmetic operators (+
, -
, *
, /
, %
and so forth) detect and disallow value overflow, to avoid unexpected results when working with numbers that become larger or smaller than the allowed value range of the type that stores them. You can opt in to value overflow behavior by using Swift’s overflow operators, as described in Overflow Operators.
Swift は、C などの言語ですでにご存知の演算子をサポートし、一般的なコーディング エラーを排除するためにいくつかの機能を改善します。 代入演算子 (=
) は、等価演算子 (==
) が意図されている場合に誤って使用されるのを防ぐため、値を返しません。 算術演算子 (+、-、*、/、%
など) は値のオーバーフローを検出して禁止し、数値を格納する型の許容値範囲より大きくなったり小さくなったりする数値を扱うときに予期しない結果が生じるのを防ぎます。 「オーバーフロー演算子」で説明されているように、Swift のオーバーフロー演算子を使用して、値のオーバーフロー動作をオプトインできます。
Swift also provides range operators that aren’t found in C, such as a..<b
and a...b
, as a shortcut for expressing a range of values.
Swift では、値の範囲を表現するためのショートカットとして、C にはない範囲演算子 (
やa..<b
など) も提供しています。a...b
This chapter describes the common operators in Swift. Advanced Operators covers Swift’s advanced operators, and describes how to define your own custom operators and implement the standard operators for your own custom types.
この章では、Swift の一般的な演算子について説明します。 「高度な演算子」では、Swift の高度な演算子をカバーし、独自のカスタム演算子を定義し、独自のカスタム型の標準演算子を実装する方法について説明します。
Terminology
用語
Operators are unary, binary, or ternary:
演算子は単項、二項、または三項です。
・Unary operators operate on a single target (such as -a
). Unary prefix operators appear immediately before their target (such as !b
), and unary postfix operators appear immediately after their target (such as c!
).
・単項演算子は単一のターゲットで動作します (-a
など)。 単項前置演算子はターゲットの直前に表示され (!b
など)、単項後置演算子はターゲットの直後に表示されます (c!
など)。
・Binary operators operate on two targets (such as 2 + 3
) and are infix because they appear in between their two targets.
・二項演算子は 2 つのターゲット (2 + 3
など) を操作し、2 つのターゲットの間に現れるため中置演算子です。
・Ternary operators operate on three targets. Like C, Swift has only one ternary operator, the ternary conditional operator (a ? b : c
).
・三項演算子は 3 つのターゲットを操作します。 C と同様、Swift には三項演算子が 1 つだけあり、それは三項条件演算子 (a ? b : c
) です。
The values that operators affect are operands. In the expression 1 + 2
, the +
symbol is an infix operator and its two operands are the values 1
and 2
.
演算子が影響する値はオペランドです。 式 1 + 2
では、+ 記号は中置演算子で、その 2 つのオペランドは値 1
と 2
です。
Assignment Operator
代入演算子
The assignment operator (a = b
) initializes or updates the value of a
with the value of b
:
代入演算子 (a = b
) は、a
の値を b
の値で初期化または更新します。
let b = 10
var a = 5
a = b
// a is now equal to 10
If the right side of the assignment is a tuple with multiple values, its elements can be decomposed into multiple constants or variables at once:
代入の右側が複数の値を持つタプルである場合、その要素は一度に複数の定数または変数に分解できます。
let (x, y) = (1, 2)
// x is equal to 1, and y is equal to 2
Unlike the assignment operator in C and Objective-C, the assignment operator in Swift doesn’t itself return a value. The following statement isn’t valid:
C や Objective-C の代入演算子とは異なり、Swift の代入演算子自体は値を返しません。 次の文は無効です。
if x = y {
// This isn't valid, because x = y doesn't return a value.
}
This feature prevents the assignment operator (=
) from being used by accident when the equal to operator (==
) is actually intended. By making if x = y
invalid, Swift helps you to avoid these kinds of errors in your code.
この機能により、実際には等号演算子 (==
) が意図されているときに、代入演算子 (=
) が誤って使用されるのを防ぎます。 if x = y
を無効にすることで、Swift はコード内でのこの種のエラーを回避できます。
Arithmetic Operators
算術演算子
Swift supports the four standard arithmetic operators for all number types:
Swift は、すべての数値タイプに対して 4 つの標準算術演算子をサポートしています。
・Addition (+
)
・加算(+
)
・Subtraction (-
)
・減算(-
)
・Multiplication (*
)
・乗算(*
)
・Division (/
)
・分割 (/
)
1 + 2 // equals 3
5 - 3 // equals 2
2 * 3 // equals 6
10.0 / 2.5 // equals 4.0
Unlike the arithmetic operators in C and Objective-C, the Swift arithmetic operators don’t allow values to overflow by default. You can opt in to value overflow behavior by using Swift’s overflow operators (such as a &+ b
). See Overflow Operators.
C や Objective-C の算術演算子とは異なり、Swift の算術演算子はデフォルトで値のオーバーフローを許可しません。 Swift のオーバーフロー演算子 (a &+ b
など) を使用して、値のオーバーフロー動作をオプトインできます。 「オーバーフロー演算子」を参照してください。
The addition operator is also supported for String
concatenation:
加算演算子はString
連結でもサポートされています。
"hello, " + "world" // equals "hello, world"
Remainder Operator
剰余演算子
The remainder operator (a % b
) works out how many multiples of b
will fit inside a
and returns the value that’s left over (known as the remainder).
剰余演算子 (a % b
) は、a
の中に b
の倍数がいくつ収まるかを計算し、残った値 (剰余と呼ばれます) を返します。
Note
注釈
The remainder operator (%
) is also known as a modulo operator in other languages. However, its behavior in Swift for negative numbers means that, strictly speaking, it’s a remainder rather than a modulo operation.
剰余演算子 (%
) は、他の言語ではモジュロ演算子としても知られています。 ただし、負の数に対する Swift の動作は、厳密に言えば、モジュロ演算ではなく剰余であることを意味します。
Here’s how the remainder operator works. To calculate 9 % 4
, you first work out how many 4
s will fit inside 9
:
剰余演算子の仕組みは次のとおりです。 9 % 4
を計算するには、まず 9
の中に 4
がいくつ入るかを計算します。
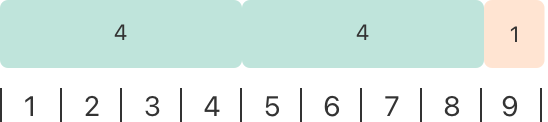
You can fit two 4
s inside 9
, and the remainder is 1
(shown in orange).
9
の中に 4
が 2 つ収まり、余りは 1
になります (オレンジ色で表示)。
In Swift, this would be written as:
Swift では、これは次のように記述されます。
9 % 4 // equals
To determine the answer for a % b
, the %
operator calculates the following equation and returns remainder
as its output:
a % b
の答えを決定するために、%
演算子は次の方程式を計算し、その出力として剰余を返します。
a
= (b
x some multiplier
) + remainder
where some multiplier
is the largest number of multiples of b
that will fit inside a
.
ここで、乗数は、a
内に収まる b
の倍数の最大数です。
Inserting 9
and 4
into this equation yields:
この式に 9
と 4
を挿入すると、次のようになります。
9
= (4
x 2
) + 1
The same method is applied when calculating the remainder for a negative value of a
:
a
の負の値の剰余を計算するときにも同じ方法が適用されます。
-9 % 4 // equals -1
Inserting -9
and 4
into the equation yields:
方程式に -9
と 4
を挿入すると、次のようになります。
-9
= (4
x -2
) + -1
giving a remainder value of -1
.
剰余値は -1
になります。
The sign of b
is ignored for negative values of b
. This means that a % b
and a % -b
always give the same answer.
b
の負の値の場合、b
の符号は無視されます。 これは、% b
と % -b
は常に同じ答えを与えることを意味します。
Unary Minus Operator
単項マイナス演算子
The sign of a numeric value can be toggled using a prefixed -
, known as the unary minus operator:
数値の符号は、単項マイナス演算子として知られる接頭辞 -
を使用して切り替えることができます。
let three = 3
let minusThree = -three // minusThree equals -3
let plusThree = -minusThree // plusThree equals 3, or "minus minus three"
The unary minus operator (-
) is prepended directly before the value it operates on, without any white space.
単項マイナス演算子 (-
) は、空白スペースを入れずに、演算対象となる値の直前に付加されます。
Unary Plus Operator
単項プラス演算子
The unary plus operator (+
) simply returns the value it operates on, without any change:
単項プラス演算子 (+
) は、演算対象の値をそのまま返します。
let minusSix = -6
let alsoMinusSix = +minusSix // alsoMinusSix equals -6
Although the unary plus operator doesn’t actually do anything, you can use it to provide symmetry in your code for positive numbers when also using the unary minus operator for negative numbers.
単項プラス演算子は実際には何も行いませんが、負の数に対して単項マイナス演算子を使用するときに、これを使用して正の数値に対してコード内で対称性を提供することができます。
Compound Assignment Operators
複合代入演算子
Like C, Swift provides compound assignment operators that combine assignment (=
) with another operation. One example is the addition assignment operator (+=
):
C と同様、Swift には代入 (=
) と別の演算を組み合わせる複合代入演算子が用意されています。 一例として、加算代入演算子 (+=
) があります。
var a = 1
a += 2
// a is now equal to 3
The expression a += 2
is shorthand for a = a + 2
. Effectively, the addition and the assignment are combined into one operator that performs both tasks at the same time.
式「a += 2
」は、a = a + 2
の短縮形です。事実上、加算と代入は両方のタスクを同時に実行する 1 つの演算子に結合されます。
Note
注釈
The compound assignment operators don’t return a value. For example, you can’t write let b = a += 2
.
複合代入演算子は値を返しません。 たとえば、let b = a += 2
と書くことはできません。
For information about the operators provided by the Swift standard library, see Operator Declarations(Link:developer.apple.com).
Swift 標準ライブラリで提供される演算子については、「演算子の宣言(Link:developer.apple.com)(英語)」を参照してください。
Comparison Operators
比較演算子
Swift supports the following comparison operators:
Swift は次の比較演算子をサポートしています。
- Equal to (
a == b
) - (
a == b
) に等しい - Not equal to (
a != b
) - (
a != b
) に等しくない - Greater than (
a > b
) - より大きい (
a > b
) - Less than (
a < b
) - 未満 (
a < b
) - Greater than or equal to (
a >= b
) - 以上 (
a >= b
) - Less than or equal to (
a <= b
) - 以下 (
a <= b
)
Note
注釈
Swift also provides two identity operators (===
and !==
), which you use to test whether two object references both refer to the same object instance. For more information, see Identity Operators.
Swift には 2 つのアイデンティティ演算子 (===
と !==
) も用意されており、2 つのオブジェクト参照が両方とも同じオブジェクト インスタンスを参照しているかどうかをテストするために使用します。 詳細については、「ID オペレーター」を参照してください。
Each of the comparison operators returns a Bool
value to indicate whether or not the statement is true:
各比較演算子は、ステートメントが true かどうかを示すBool
値を返します。
1 == 1 // true because 1 is equal to 1
2 != 1 // true because 2 isn't equal to 1
2 > 1 // true because 2 is greater than 1
1 < 2 // true because 1 is less than 2
1 >= 1 // true because 1 is greater than or equal to 1
2 <= 1 // false because 2 isn't less than or equal to 1
Comparison operators are often used in conditional statements, such as the if
statement:
比較演算子は、if
ステートメントなどの条件ステートメントでよく使用されます。
let name = "world"
if name == "world" {
print("hello, world")
} else {
print("I'm sorry \(name), but I don't recognize you")
}
// Prints "hello, world", because name is indeed equal to "world".
For more about the if
statement, see Control Flow.
if ステートメントの詳細については、「制御フロー」を参照してください。
You can compare two tuples if they have the same type and the same number of values. Tuples are compared from left to right, one value at a time, until the comparison finds two values that aren’t equal. Those two values are compared, and the result of that comparison determines the overall result of the tuple comparison. If all the elements are equal, then the tuples themselves are equal. For example:
2 つのタプルの型と値の数が同じであれば、それらを比較できます。 タプルは、等しくない 2 つの値が見つかるまで、一度に 1 つの値を左から右に比較します。 これら 2 つの値が比較され、その比較の結果によってタプル比較の全体的な結果が決まります。 すべての要素が等しい場合、タプル自体も等しいことになります。 例えば:
(1, "zebra") < (2, "apple") // true because 1 is less than 2; "zebra" and "apple" aren't compared
(3, "apple") < (3, "bird") // true because 3 is equal to 3, and "apple" is less than "bird"
(4, "dog") == (4, "dog") // true because 4 is equal to 4, and "dog" is equal to "dog"
In the example above, you can see the left-to-right comparison behavior on the first line. Because 1
is less than 2
, (1, "zebra")
is considered less than (2, "apple")
, regardless of any other values in the tuples. It doesn’t matter that "zebra"
isn’t less than "apple"
, because the comparison is already determined by the tuples’ first elements. However, when the tuples’ first elements are the same, their second elements are compared — this is what happens on the second and third line.
上の例では、最初の行で左から右への比較動作が確認できます。 1
は 2
より小さいため、タプル内の他の値に関係なく、(1, "zebra")
は (2, "apple")
より小さいとみなされます。 "zebra"
が"apple"
より小さくないことは問題ではありません。比較はタプルの最初の要素によってすでに決定されているためです。 ただし、タプルの最初の要素が同じ場合、2 番目の要素が比較されます。これが 2 行目と 3 行目で行われます。
Tuples can be compared with a given operator only if the operator can be applied to each value in the respective tuples. For example, as demonstrated in the code below, you can compare two tuples of type (String, Int)
because both String
and Int
values can be compared using the <
operator. In contrast, two tuples of type (String, Bool)
can’t be compared with the <
operator because the <
operator can’t be applied to Bool
values.
タプルは、その演算子がそれぞれのタプルの各値に適用できる場合にのみ、その演算子と比較できます。 たとえば、以下のコードに示すように、<
演算子を使用して String
値と Int
値の両方を比較できるため、型 (String, Int)
の 2 つのタプルを比較できます。 対照的に、(String, Bool)
型の 2 つのタプルは、<
演算子と比較できません。これは、<
演算子を Bool
値に適用できないためです。
("blue", -1) < ("purple", 1) // OK, evaluates to true
("blue", false) < ("purple", true) // Error because < can't compare Boolean values
Note
注釈
The Swift standard library includes tuple comparison operators for tuples with fewer than seven elements. To compare tuples with seven or more elements, you must implement the comparison operators yourself.
Swift 標準ライブラリには、要素が 7 つ未満のタプル用のタプル比較演算子が含まれています。 7 つ以上の要素を含むタプルを比較するには、比較演算子を自分で実装する必要があります。
Ternary Conditional Operator
3項条件演算子
The ternary conditional operator is a special operator with three parts, which takes the form question ? answer1 : answer2
. It’s a shortcut for evaluating one of two expressions based on whether question
is true or false. If question
is true, it evaluates answer1
and returns its value; otherwise, it evaluates answer2
and returns its value.
3 項条件演算子は、3 つの部分からなる特別な演算子で、質問の形式を取ります。 question ? answer1 : answer2
. これは、question
が真か偽かに基づいて 2 つの式のうちの 1 つを評価するためのショートカットです。 question
がtrueの場合、answer1
を評価してその値を返します。 それ以外の場合は、answer2
を評価し、その値を返します。
The ternary conditional operator is shorthand for the code below:
3 項条件演算子は、以下のコードの短縮形です。
if question {
answer1
} else {
answer2
}
Here’s an example, which calculates the height for a table row. The row height should be 50 points taller than the content height if the row has a header, and 20 points taller if the row doesn’t have a header:
以下は、テーブルの行の高さを計算する例です。 行にヘッダーがある場合、行の高さはコンテンツの高さより 50 ポイント高く、行にヘッダーがない場合は 20 ポイント高くする必要があります。
let contentHeight = 40
let hasHeader = true
let rowHeight = contentHeight + (hasHeader ? 50 : 20)
// rowHeight is equal to 90
The example above is shorthand for the code below:
上記の例は、以下のコードの短縮形です。
let contentHeight = 40
let hasHeader = true
let rowHeight: Int
if hasHeader {
rowHeight = contentHeight + 50
} else {
rowHeight = contentHeight + 20
}
// rowHeight is equal to 90
The first example’s use of the ternary conditional operator means that rowHeight
can be set to the correct value on a single line of code, which is more concise than the code used in the second example.
最初の例では三項条件演算子を使用していますが、これは 1 行のコードで rowHeight
を正しい値に設定できることを意味しており、2 番目の例で使用されているコードよりも簡潔です。
The ternary conditional operator provides an efficient shorthand for deciding which of two expressions to consider. Use the ternary conditional operator with care, however. Its conciseness can lead to hard-to-read code if overused. Avoid combining multiple instances of the ternary conditional operator into one compound statement.
3 項条件演算子は、2 つの式のうちどちらを考慮するかを決定するための効率的な省略表現を提供します。 ただし、三項条件演算子は注意して使用してください。 その簡潔さは、使いすぎるとコードが読みにくくなる可能性があります。 三項条件演算子の複数のインスタンスを 1 つの複合ステートメントに結合することは避けてください。
Nil-Coalescing Operator
ニル合体演算子
The nil-coalescing operator (a ?? b
) unwraps an optional a
if it contains a value, or returns a default value b
if a
is nil
. The expression a
is always of an optional type. The expression b
must match the type that’s stored inside a
.
nil合体演算子(a ?? b
)は、値が含まれている場合はオプションのa
をアンラップし、a
がnil
の場合はデフォルト値b
を返します。 式 a
は常にオプションのタイプです。 式 b
は、a
内に格納されている型と一致する必要があります。
The nil-coalescing operator is shorthand for the code below:
nil合体演算子は、以下のコードの短縮形です。
a != nil ? a! : b
The code above uses the ternary conditional operator and forced unwrapping (a!
) to access the value wrapped inside a
when a
isn’t nil
, and to return b
otherwise. The nil-coalescing operator provides a more elegant way to encapsulate this conditional checking and unwrapping in a concise and readable form.
上記のコードは、三項条件演算子と強制アンラップ (a!
) を使用して、a
が nil
でない場合は a
内にラップされた値にアクセスし、それ以外の場合は b
を返します。 nil合体演算子は、この条件付きチェックとアンラップを簡潔で読みやすい形式でカプセル化する、より洗練された方法を提供します。
Note
注釈
If the value of a
is non-nil
, the value of b
isn’t evaluated. This is known as short-circuit evaluation.
a
の値が非 nil
の場合、b
の値は評価されません。 これは短絡評価として知られています。
The example below uses the nil-coalescing operator to choose between a default color name and an optional user-defined color name:
以下の例では、nil-coalescing 演算子を使用して、デフォルトの色名とオプションのユーザー定義の色名のどちらかを選択します。
let defaultColorName = "red"
var userDefinedColorName: String? // defaults to nil
var colorNameToUse = userDefinedColorName ?? defaultColorName
// userDefinedColorName is nil, so colorNameToUse is set to the default of "red"
The userDefinedColorName
variable is defined as an optional String
, with a default value of nil
. Because userDefinedColorName
is of an optional type, you can use the nil-coalescing operator to consider its value. In the example above, the operator is used to determine an initial value for a String
variable called colorNameToUse
. Because userDefinedColorName
is nil
, the expression userDefinedColorName ?? defaultColorName
returns the value of defaultColorName
, or "red"
.
userDefinedColorName
変数はオプションのString
として定義され、デフォルト値は nil
です。 userDefinedColorNam
e はオプションのタイプであるため、nil-coalescing 演算子を使用してその値を検討できます。 上の例では、演算子は colorNameToUse
というString
変数の初期値を決定するために使用されています。 userDefinedColorName
が nil
であるため、式 userDefinedColorName ?? defaultColorName
は、defaultColorName
の値、つまり「赤」を返します。
If you assign a non-nil
value to userDefinedColorName
and perform the nil-coalescing operator check again, the value wrapped inside userDefinedColorName
is used instead of the default:
userDefinedColorName
に nil
以外の値を割り当て、nil
合体演算子チェックを再度実行すると、userDefinedColorName
内にラップされた値がデフォルトの代わりに使用されます。
userDefinedColorName = "green"
colorNameToUse = userDefinedColorName ?? defaultColorName
// userDefinedColorName isn't nil, so colorNameToUse is set to "green"
Range Operators
範囲演算子
Swift includes several range operators, which are shortcuts for expressing a range of values.
Swift には、値の範囲を表現するためのショートカットである範囲演算子がいくつか含まれています。
Closed Range Operator
クローズドレンジオペレーター
The closed range operator (a...b
) defines a range that runs from a
to b
, and includes the values a
and b
. The value of a
must not be greater than b
.
閉範囲演算子(a…b
)は、a
から b
までの範囲を定義し、値 a
と b
を含みます。 a
の値は b
より大きくてはなりません。
The closed range operator is useful when iterating over a range in which you want all of the values to be used, such as with a for
–in
loop:
閉範囲演算子は、for-in
ループなど、すべての値を使用する範囲を反復処理する場合に便利です。
for index in 1...5 {
print("\(index) times 5 is \(index * 5)")
}
// 1 times 5 is 5
// 2 times 5 is 10
// 3 times 5 is 15
// 4 times 5 is 20
// 5 times 5 is 25
For more about for
–in
loops, see Control Flow.
for-in ループの詳細については、「制御フロー」を参照してください。
Half-Open Range Operator
半開範囲演算子
The half-open range operator (a..<b
) defines a range that runs from a
to b
, but doesn’t include b
. It’s said to be half-open because it contains its first value, but not its final value. As with the closed range operator, the value of a
must not be greater than b
. If the value of a
is equal to b
, then the resulting range will be empty.
半開範囲演算子(a..<
b)は、a
から b
までの範囲を定義しますが、b
は含まれません。 最初の値は含まれていますが、最終値は含まれていないため、ハーフオープンであると言われます。 閉範囲演算子の場合と同様、a
の値は b
より大きくてはなりません。 a
の値が b
に等しい場合、結果の範囲は空になります。
Half-open ranges are particularly useful when you work with zero-based lists such as arrays, where it’s useful to count up to (but not including) the length of the list:
ハーフオープン範囲は、配列などの 0 から始まるリストを操作する場合に特に便利です。リストの長さまで (ただし、リストの長さは含まない) カウントすると便利です。
let names = ["Anna", "Alex", "Brian", "Jack"]
let count = names.count
for i in 0..<count {
print("Person \(i + 1) is called \(names[i])")
}
// Person 1 is called Anna
// Person 2 is called Alex
// Person 3 is called Brian
// Person 4 is called Jack
Note that the array contains four items, but 0..<count
only counts as far as 3
(the index of the last item in the array), because it’s a half-open range. For more about arrays, see Arrays.
配列には 4 つの項目が含まれていますが、0..<count
は半オープン範囲であるため、3
(配列内の最後の項目のインデックス) までしかカウントされないことに注意してください。 配列の詳細については、「配列」をご覧ください。
One-Sided Ranges
片側範囲
The closed range operator has an alternative form for ranges that continue as far as possible in one direction — for example, a range that includes all the elements of an array from index 2 to the end of the array. In these cases, you can omit the value from one side of the range operator. This kind of range is called a one-sided range because the operator has a value on only one side. For example:
閉範囲演算子には、一方向に可能な限り続く範囲の代替形式があります。たとえば、インデックス 2 から配列の末尾までの配列のすべての要素を含む範囲などです。 このような場合、範囲演算子の片側の値を省略できます。 この種の範囲は、演算子が片側のみに値を持つため、片側範囲と呼ばれます。 例えば:
for name in names[2...] {
print(name)
}
// Brian
// Jack
for name in names[...2] {
print(name)
}
// Anna
// Alex
// Brian
The half-open range operator also has a one-sided form that’s written with only its final value. Just like when you include a value on both sides, the final value isn’t part of the range. For example:
半開範囲演算子には、最終値のみを記述した片側形式もあります。 両側に値を含める場合と同様に、最終値は範囲の一部ではありません。 例えば:
for name in names[..<2] {
print(name)
}
// Anna
// Alex
One-sided ranges can be used in other contexts, not just in subscripts. You can’t iterate over a one-sided range that omits a first value, because it isn’t clear where iteration should begin. You can iterate over a one-sided range that omits its final value; however, because the range continues indefinitely, make sure you add an explicit end condition for the loop. You can also check whether a one-sided range contains a particular value, as shown in the code below.
片側範囲は、添字だけでなく他のコンテキストでも使用できます。 反復をどこから開始すべきかが明確でないため、最初の値を省略する片側範囲を反復することはできません。 最終値を省略した片側範囲を反復処理できます。 ただし、範囲は無限に続くため、ループには必ず明示的な終了条件を追加してください。 以下のコードに示すように、片側範囲に特定の値が含まれているかどうかを確認することもできます。
let range = ...5
range.contains(7) // false
range.contains(4) // true
range.contains(-1) // true
Logical Operators
論理演算子
Logical operators modify or combine the Boolean logic values true
and false
. Swift supports the three standard logical operators found in C-based languages:
論理演算子は、ブール論理値 true
と fals
e を変更または組み合わせます。 Swift は、C ベースの言語にある 3 つの標準論理演算子をサポートしています。
- Logical NOT (
!a
) - 論理否定 (
!a
) - Logical AND (
a && b
) - 論理積 (
a && b
) - Logical OR (
a || b
) - 論理和 (
a || b
)
Logical NOT Operator
論理否定演算子
The logical NOT operator (!a
) inverts a Boolean value so that true
becomes false
, and false
becomes true
.
論理 NOT 演算子 (!a
) はブール値を反転して、true
が false
になり、false
が true
になります。
The logical NOT operator is a prefix operator, and appears immediately before the value it operates on, without any white space. It can be read as “not a
”, as seen in the following example:
論理 NOT 演算子は接頭辞演算子であり、演算対象となる値の直前に空白なしで現れます。 次の例に示すように、「not a
」と読むこともできます。
let allowedEntry = false
if !allowedEntry {
print("ACCESS DENIED")
}
// Prints "ACCESS DENIED"
The phrase if !allowedEntry
can be read as “if not allowed entry.” The subsequent line is only executed if “not allowed entry” is true; that is, if allowedEntry
is false
.
if !allowedEntry
というフレーズは、「エントリが許可されていない場合」と読むことができます。 後続の行は、「エントリを許可しない」が true
の場合にのみ実行されます。 つまり、allowedEntry
が false
の場合です。
As in this example, careful choice of Boolean constant and variable names can help to keep code readable and concise, while avoiding double negatives or confusing logic statements.
この例のように、ブール定数名と変数名を慎重に選択すると、二重否定や混乱を招く論理ステートメントを避けながら、コードを読みやすく簡潔に保つことができます。
Logical AND Operator
論理積演算子
The logical AND operator (a && b
) creates logical expressions where both values must be true
for the overall expression to also be true
.
論理 AND 演算子 (a && b
) は、式全体が true
になるためには両方の値が true
でなければならない論理式を作成します。
If either value is false
, the overall expression will also be false
. In fact, if the first value is false
, the second value won’t even be evaluated, because it can’t possibly make the overall expression equate to true
. This is known as short-circuit evaluation.
いずれかの値が false
の場合、式全体も false
になります。 実際、最初の値が false
の場合、2 番目の値は評価すらされません。これは、式全体を true
とみなすことができないためです。 これは短絡評価として知られています。
This example considers two Bool
values and only allows access if both values are true
:
この例では 2 つのBool
を考慮し、両方の値が true
の場合にのみアクセスを許可します。
let enteredDoorCode = true
let passedRetinaScan = false
if enteredDoorCode && passedRetinaScan {
print("Welcome!")
} else {
print("ACCESS DENIED")
}
// Prints "ACCESS DENIED"
Logical OR Operator
論理和演算子
The logical OR operator (a || b
) is an infix operator made from two adjacent pipe characters. You use it to create logical expressions in which only one of the two values has to be true
for the overall expression to be true
.
論理 OR 演算子 (a || b
) は、2 つの隣接するパイプ文字から構成される中置演算子です。 これを使用して、式全体が true
になるために 2 つの値のうち 1 つだけが true
でなければならない論理式を作成します。
Like the Logical AND operator above, the Logical OR operator uses short-circuit evaluation to consider its expressions. If the left side of a Logical OR expression is true
, the right side isn’t evaluated, because it can’t change the outcome of the overall expression.
上記の論理 AND
演算子と同様に、論理 OR
演算子は短絡評価を使用して式を検討します。 論理 OR
式の左側が true
の場合、式全体の結果を変更できないため、右側は評価されません。
In the example below, the first Bool
value (hasDoorKey
) is false
, but the second value (knowsOverridePassword
) is true
. Because one value is true
, the overall expression also evaluates to true
, and access is allowed:
以下の例では、最初のブール値 (hasDoorKey
) は false
ですが、2 番目の値 (knowsOverridePassword
) は true
です。 1 つの値が true
であるため、式全体も true
と評価され、アクセスが許可されます。
let hasDoorKey = false
let knowsOverridePassword = true
if hasDoorKey || knowsOverridePassword {
print("Welcome!")
} else {
print("ACCESS DENIED")
}
// Prints "Welcome!"
Combining Logical Operators
論理演算子の結合
You can combine multiple logical operators to create longer compound expressions:
複数の論理演算子を組み合わせて、より長い複合式を作成できます。
if enteredDoorCode && passedRetinaScan || hasDoorKey || knowsOverridePassword {
print("Welcome!")
} else {
print("ACCESS DENIED")
}
// Prints "Welcome!"
This example uses multiple &&
and ||
operators to create a longer compound expression. However, the &&
and ||
operators still operate on only two values, so this is actually three smaller expressions chained together. The example can be read as:
この例では、複数の &&
と ||
を使用しています。 演算子を使用して、より長い複合式を作成します。 ただし、&&
と ||
演算子は依然として 2 つの値のみを操作するため、これは実際には 3 つの小さな式が連鎖したものになります。 この例は次のように解釈できます。
If we’ve entered the correct door code and passed the retina scan, or if we have a valid door key, or if we know the emergency override password, then allow access.
正しいドア コードを入力して網膜スキャンに合格した場合、または有効なドア キーを持っている場合、または緊急時オーバーライド パスワードを知っている場合は、アクセスを許可します。
Based on the values of enteredDoorCode
, passedRetinaScan
, and hasDoorKey
, the first two subexpressions are false
. However, the emergency override password is known, so the overall compound expression still evaluates to true
.
enteredDoorCode
、passedRetinaScan
、hasDoorKey
の値に基づくと、最初の 2 つの部分式は false
です。 ただし、緊急時オーバーライド パスワードはわかっているため、複合式全体は依然として true
と評価されます。
Note
注釈
The Swift logical operators &&
and ||
are left-associative, meaning that compound expressions with multiple logical operators evaluate the leftmost subexpression first.
Swift 論理演算子 &&
および ||
これは、複数の論理演算子を含む複合式が最初に左端の部分式を評価することを意味します。
Explicit Parentheses
明示的な括弧
It’s sometimes useful to include parentheses when they’re not strictly needed, to make the intention of a complex expression easier to read. In the door access example above, it’s useful to add parentheses around the first part of the compound expression to make its intent explicit:
厳密には必要ではない場合でも、複雑な式の意図を読みやすくするために、括弧を含めると便利な場合があります。 上記のドア アクセスの例では、複合式の最初の部分にかっこを追加して、その意図を明示すると便利です。
if (enteredDoorCode && passedRetinaScan) || hasDoorKey || knowsOverridePassword {
print("Welcome!")
} else {
print("ACCESS DENIED")
}
// Prints "Welcome!"
The parentheses make it clear that the first two values are considered as part of a separate possible state in the overall logic. The output of the compound expression doesn’t change, but the overall intention is clearer to the reader. Readability is always preferred over brevity; use parentheses where they help to make your intentions clear.
括弧は、最初の 2 つの値がロジック全体の別の可能な状態の一部として考慮されることを明確にします。 複合式の出力は変わりませんが、全体的な意図が読者にとってより明確になります。 簡潔さよりも読みやすさが常に優先されます。 意図を明確にするために括弧を使用してください。
Beta Software
ベータ版ソフトウェアー
This documentation contains preliminary information about an API or technology in development. This information is subject to change, and software implemented according to this documentation should be tested with final operating system software.
このドキュメントには、開発中の API またはテクノロジに関する予備情報が含まれています。 この情報は変更される可能性があり、このドキュメントに従って実装されたソフトウェアは、最終的なオペレーティング システム ソフトウェアでテストする必要があります。
Learn more about using Apple’s beta software(Link:developer.apple.com).
詳しくは、Apple のベータ版ソフトウェア(Link:developer.apple.com)(英語)の使用についてをご覧ください。