下記URLから引用し、日本語訳をつけてみました。
https://docs.swift.org/swift-book/documentation/the-swift-programming-language/collectiontypes/
Organize data using arrays, sets, and dictionaries.
配列、セット、辞書を使用してデータを整理します。
Swift provides three primary collection types, known as arrays, sets, and dictionaries, for storing collections of values. Arrays are ordered collections of values. Sets are unordered collections of unique values. Dictionaries are unordered collections of key-value associations.
Swift は、値のコレクションを保存するために、配列、セット、辞書と呼ばれる 3 つの主要なコレクション 型を提供します。 配列は、順序付けられた値のコレクションです。 セットは、順序付けされていない一意の値のコレクションです。 ディクショナリは、キーと値の関連付けの順序付けされていないコレクションです。
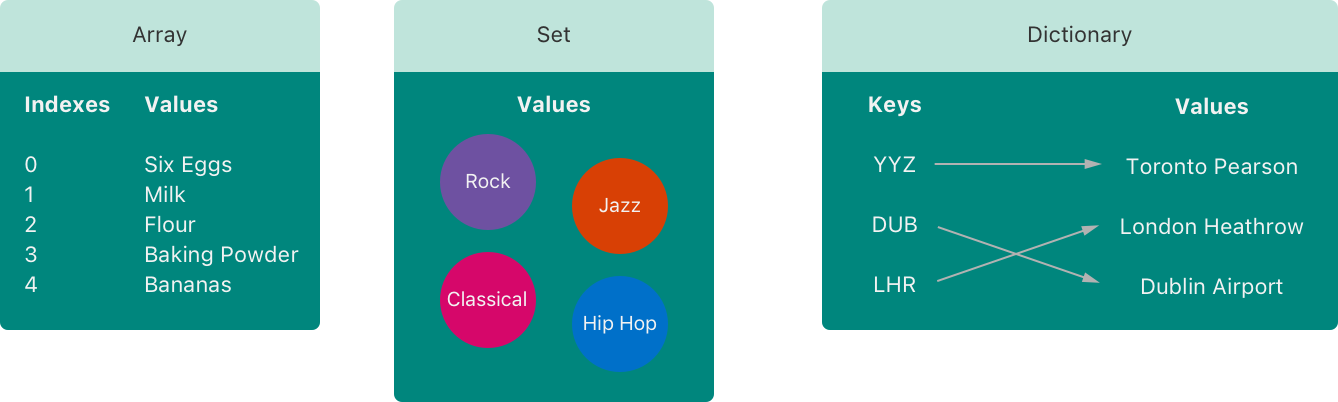
Arrays, sets, and dictionaries in Swift are always clear about the types of values and keys that they can store. This means that you can’t insert a value of the wrong type into a collection by mistake. It also means you can be confident about the type of values you will retrieve from a collection.
Swift の配列、セット、辞書は、格納できる値とキーの種類を常に明確にしています。 これは、誤って間違った型の値をコレクションに挿入することができないことを意味します。 これは、コレクションから取得する値の型について確信を持てることも意味します。
Note
注釈
Swift’s array, set, and dictionary types are implemented as generic collections. For more about generic types and collections, see Generics.
Swift の配列、セット、辞書型は汎用コレクションとして実装されます。 ジェネリック型とコレクションの詳細については、「ジェネリック」を参照してください。
Mutability of Collections
コレクションの可変性
If you create an array, a set, or a dictionary, and assign it to a variable, the collection that’s created will be mutable. This means that you can change (or mutate) the collection after it’s created by adding, removing, or changing items in the collection. If you assign an array, a set, or a dictionary to a constant, that collection is immutable, and its size and contents can’t be changed.
配列、セット、またはディクショナリを作成し、それを変数に割り当てると、作成されたコレクションは変更可能になります。 これは、コレクションの作成後に、コレクション内の項目を追加、削除、または変更することでコレクションを変更できることを意味します。 配列、セット、またはディクショナリを定数に割り当てると、そのコレクションは不変となり、そのサイズと内容は変更できません。
Note
注釈
It’s good practice to create immutable collections in all cases where the collection doesn’t need to change. Doing so makes it easier for you to reason about your code and enables the Swift compiler to optimize the performance of the collections you create.
コレクションを変更する必要がないすべての場合に、不変のコレクションを作成することをお勧めします。 そうすることで、コードの推論が容易になり、Swift コンパイラーが作成したコレクションのパフォーマンスを最適化できるようになります。
Arrays
配列
An array stores values of the same type in an ordered list. The same value can appear in an array multiple times at different positions.
配列は、同じ型の値を順序付きリストに格納します。 同じ値が配列内の異なる位置に複数回現れることがあります。
Note
注釈
Swift’s Array
type is bridged to Foundation’s NSArray
class.
Swift の Array
型は 基本の NSArray
クラスに橋渡しされます。
For more information about using Array
with Foundation and Cocoa, see Bridging Between Array and NSArray(Link:developer.apple.com).
基本 および Cocoa
で Array
を使用する方法の詳細については、「Array と NSArray 間の橋渡し(Link:developer.apple.com)(英語)」を参照してください。
Array Type Shorthand Syntax
配列型の短縮構文
The type of a Swift array is written in full as Array<Element>
, where Element
is the type of values the array is allowed to store. You can also write the type of an array in shorthand form as [Element]
. Although the two forms are functionally identical, the shorthand form is preferred and is used throughout this guide when referring to the type of an array.
Swift 配列の型は完全に Array<Element>
として記述されます。Element
は配列に保存できる値の型です。 配列の型を [Element]
という省略形で記述することもできます。 2 つの形式は機能的には同じですが、配列の型を参照する場合は省略形が好まれ、このガイド全体で使用されます。
Creating an Empty Array
空の配列の作成
You can create an empty array of a certain type using initializer syntax:
イニシャライザ構文を使用して、特定の型の空の配列を作成できます。
var someInts: [Int] = []
print("someInts is of type [Int] with \(someInts.count) items.")
// Prints "someInts is of type [Int] with 0 items."
Note that the type of the someInts
variable is inferred to be [Int]
from the type of the initializer.
someInts
変数の型はイニシャライザの型から [Int]
であると推測されることに注意してください。
Alternatively, if the context already provides type information, such as a function argument or an already typed variable or constant, you can create an empty array with an empty array literal, which is written as []
(an empty pair of square brackets):
あるいは、コンテキストが関数の引数やすでに型指定された変数や定数などの型情報をすでに提供している場合は、 []
(空の角括弧のペア)として記述される空の配列リテラルを含む空の配列を作成できます。
someInts.append(3)
// someInts now contains 1 value of type Int
someInts = []
// someInts is now an empty array, but is still of type [Int]
Creating an Array with a Default Value
デフォルト値を使用した配列の作成
Swift’s Array
type also provides an initializer for creating an array of a certain size with all of its values set to the same default value. You pass this initializer a default value of the appropriate type (called repeating
): and the number of times that value is repeated in the new array (called count
):
Swift の Array
型は、すべての値が同じデフォルト値に設定された特定のサイズの配列を作成するためのイニシャライザも提供します。 このイニシャライザに適切な型のデフォルト値( repeating
と呼ばれます)を渡し、その値が新しい配列で繰り返される回数(count
と呼ばれます)を渡します。
var threeDoubles = Array(repeating: 0.0, count: 3)
// threeDoubles is of type [Double], and equals [0.0, 0.0, 0.0]
Creating an Array by Adding Two Arrays Together
2 つの配列を加算して配列を作成する
You can create a new array by adding together two existing arrays with compatible types with the addition operator (+
). The new array’s type is inferred from the type of the two arrays you add together:
新しい配列を作成するには、互換性のある型を持つ 2 つの既存の配列を加算演算子 (+
) を使用して加算します。 新しい配列の型は、加算した 2 つの配列の型から推測されます。
var anotherThreeDoubles = Array(repeating: 2.5, count: 3)
// anotherThreeDoubles is of type [Double], and equals [2.5, 2.5, 2.5]
var sixDoubles = threeDoubles + anotherThreeDoubles
// sixDoubles is inferred as [Double], and equals [0.0, 0.0, 0.0, 2.5, 2.5, 2.5]
Creating an Array with an Array Literal
配列リテラルを使用した配列の作成
You can also initialize an array with an array literal, which is a shorthand way to write one or more values as an array collection. An array literal is written as a list of values, separated by commas, surrounded by a pair of square brackets:
配列リテラルを使用して配列を初期化することもできます。これは、1 つ以上の値を配列コレクションとして書き込む簡単な方法です。 配列リテラルは、カンマで区切られ、角かっこで囲まれた値のリストとして記述されます。
[<#value 1#>, <#value 2#>, <#value 3#>]
The example below creates an array called shoppingList
to store String
values:
以下の例では、 String
値を保存するために、shoppingList
という配列を作成します。
var shoppingList: [String] = ["Eggs", "Milk"]
// shoppingList has been initialized with two initial items
The shoppingList
variable is declared as “an array of string values”, written as [String]
. Because this particular array has specified a value type of String
, it’s allowed to store String
values only. Here, the shoppingList
array is initialized with two String
values ("Eggs"
and "Milk"
), written within an array literal.
shoppingList
変数は「文字列値の配列」として宣言され、[String]
と記述されます。 この特定の配列では値の型が String
に指定されているため、String
値のみを格納できます。 ここで、shoppingList
配列は、配列リテラル内に記述された 2 つの String
値 ("Eggs"
と"Milk"
) で初期化されます。
Note
注釈
The shoppingList
array is declared as a variable (with the var
introducer) and not a constant (with the let
introducer) because more items are added to the shopping list in the examples below.
以下の例ではショッピング リストにさらにアイテムが追加されているため、shoppingList
配列は定数 (let
イントロデューサーを使用) ではなく変数 (var
イントロデューサーを使用) として宣言されています。
In this case, the array literal contains two String
values and nothing else. This matches the type of the shoppingList
variable’s declaration (an array that can only contain String
values), and so the assignment of the array literal is permitted as a way to initialize shoppingList
with two initial items.
この場合、配列リテラルには 2 つの String
値が含まれており、他には何も含まれていません。 これは、shoppingList
変数の宣言の型(String
値のみを含むことができる配列)と一致するため、2 つの初期項目で shoppingList
を初期化する方法として、配列リテラルの割り当てが許可されます。
Thanks to Swift’s type inference, you don’t have to write the type of the array if you’re initializing it with an array literal containing values of the same type. The initialization of shoppingList
could have been written in a shorter form instead:
Swift の型推論のおかげで、同じ型の値を含む配列リテラルを使用して配列を初期化する場合、配列の型を記述する必要はありません。 shoppingList
の初期化は、代わりに短い形式で記述することもできます。
var shoppingList = ["Eggs", "Milk"]
Because all values in the array literal are of the same type, Swift can infer that [String]
is the correct type to use for the shoppingList
variable.
配列リテラル内の値はすべて同じ型であるため、Swift は [String]
が shoppingList
変数に使用する正しい型であると推測できます。
Accessing and Modifying an Array
配列へのアクセスと変更
You access and modify an array through its methods and properties, or by using subscript syntax.
配列にアクセスして変更するには、配列のメソッドとプロパティを使用するか、添え字構文を使用します。
To find out the number of items in an array, check its read-only count
property:
配列内の項目数を確認するには、その読み取り専用の count
プロパティを確認します。
print("The shopping list contains \(shoppingList.count) items.")
// Prints "The shopping list contains 2 items."
Use the Boolean isEmpty
property as a shortcut for checking whether the count
property is equal to 0
:
count
プロパティが 0 に等しいかどうかを確認するためのショートカットとして、Boolean isEmpty
プロパティを使用します。
if shoppingList.isEmpty {
print("The shopping list is empty.")
} else {
print("The shopping list isn't empty.")
}
// Prints "The shopping list isn't empty."
You can add a new item to the end of an array by calling the array’s append(_:)
method:
配列の append(_:)
メソッドを呼び出すことで、配列の末尾に新しい項目を追加できます。
shoppingList.append("Flour")
// shoppingList now contains 3 items, and someone is making pancakes
Alternatively, append an array of one or more compatible items with the addition assignment operator (+=
):
あるいは、加算代入演算子 (+=
) を使用して、1 つ以上の互換性のある項目の配列を追加します。
shoppingList += ["Baking Powder"]
// shoppingList now contains 4 items
shoppingList += ["Chocolate Spread", "Cheese", "Butter"]
// shoppingList now contains 7 items
Retrieve a value from the array by using subscript syntax, passing the index of the value you want to retrieve within square brackets immediately after the name of the array:
添字構文を使用して配列から値を取得し、取得する値のインデックスを配列名の直後の角括弧内に渡します。
var firstItem = shoppingList[0]
// firstItem is equal to "Eggs"
Note
注釈
The first item in the array has an index of 0
, not 1
. Arrays in Swift are always zero-indexed.
配列の最初の項目のインデックスは 1 ではなく 0 です。Swift の配列のインデックスは常に 0 です。
You can use subscript syntax to change an existing value at a given index:
添え字構文を使用して、特定のインデックスの既存の値を変更できます。
shoppingList[0] = "Six eggs"
// the first item in the list is now equal to "Six eggs" rather than "Eggs"
When you use subscript syntax, the index you specify needs to be valid. For example, writing shoppingList[shoppingList.count] = "Salt"
to try to append an item to the end of the array results in a runtime error.
添字構文を使用する場合、指定するインデックスは有効である必要があります。 たとえば、shoppingList[shoppingList.count] = "Salt"
と記述して配列の末尾に項目を追加しようとすると、実行時エラーが発生します。
You can also use subscript syntax to change a range of values at once, even if the replacement set of values has a different length than the range you are replacing. The following example replaces "Chocolate Spread"
, "Cheese"
, and "Butter"
with "Bananas"
and "Apples"
:
置換する値のセットの長さが置換する範囲と異なる場合でも、添字構文を使用して値の範囲を一度に変更することもできます。 次の例では、「Chocolate Spread
」、「Cheese
」、「Butter
」を「Bananas
」と「Apples
」に置き換えます。
shoppingList[4...6] = ["Bananas", "Apples"]
// shoppingList now contains 6 items
To insert an item into the array at a specified index, call the array’s insert(_:at:)
method:
配列の指定されたインデックスに項目を挿入するには、配列の insert(_:at:)
メソッドを呼び出します。
shoppingList.insert("Maple Syrup", at: 0)
// shoppingList now contains 7 items
// "Maple Syrup" is now the first item in the list
This call to the insert(_:at:)
method inserts a new item with a value of "Maple Syrup"
at the very beginning of the shopping list, indicated by an index of 0
.
この insert(_:at:)
メソッドの呼び出しにより、値「メープル シロップ」を持つ新しい商品が買い物リストの先頭に挿入され、インデックス 0
で示されます。
Similarly, you remove an item from the array with the remove(at:)
method. This method removes the item at the specified index and returns the removed item (although you can ignore the returned value if you don’t need it):
同様に、remove(at:)
メソッドを使用して配列から項目を削除します。 このメソッドは、指定されたインデックスにある項目を削除し、削除された項目を返します (ただし、戻り値が必要ない場合は無視できます)。
let mapleSyrup = shoppingList.remove(at: 0)
// the item that was at index 0 has just been removed
// shoppingList now contains 6 items, and no Maple Syrup
// the mapleSyrup constant is now equal to the removed "Maple Syrup" string
Note
注釈
If you try to access or modify a value for an index that’s outside of an array’s existing bounds, you will trigger a runtime error. You can check that an index is valid before using it by comparing it to the array’s count
property. The largest valid index in an array is count - 1
because arrays are indexed from zero — however, when count
is 0
(meaning the array is empty), there are no valid indexes.
配列の既存の範囲外にあるインデックスの値にアクセスしたり変更しようとすると、実行時エラーが発生します。 インデックスを使用する前に、配列の count
プロパティと比較することで、インデックスが有効であることを確認できます。 配列のインデックスはゼロから始まるため、配列内の最大の有効なインデックスは count - 1
です。ただし、count
が 0
の場合(配列が空であることを意味します)、有効なインデックスはありません。
Any gaps in an array are closed when an item is removed, and so the value at index 0
is once again equal to "Six eggs"
:
配列内のギャップは項目が削除されると閉じられるため、インデックス 0
の値は再び"Six eggs"
と等しくなります。
firstItem = shoppingList[0]
// firstItem is now equal to "Six eggs"
If you want to remove the final item from an array, use the removeLast()
method rather than the remove(at:)
method to avoid the need to query the array’s count
property. Like the remove(at:)
method, removeLast()
returns the removed item:
配列から最後の項目を削除する場合は、remove(at:)
メソッドではなく、removeLast()
メソッドを使用して、配列の count
プロパティをクエリする必要性を回避します。 remove(at:)
メソッドと同様に、removeLast()
は削除された項目を返します。
let apples = shoppingList.removeLast()
// the last item in the array has just been removed
// shoppingList now contains 5 items, and no apples
// the apples constant is now equal to the removed "Apples" string
Iterating Over an Array
配列の反復処理
You can iterate over the entire set of values in an array with the for
–in
loop:
for-in
ループを使用して、配列内の値のセット全体を反復処理できます。
for item in shoppingList {
print(item)
}
// Six eggs
// Milk
// Flour
// Baking Powder
// Bananas
If you need the integer index of each item as well as its value, use the enumerated()
method to iterate over the array instead. For each item in the array, the enumerated()
method returns a tuple composed of an integer and the item. The integers start at zero and count up by one for each item; if you enumerate over a whole array, these integers match the items’ indices. You can decompose the tuple into temporary constants or variables as part of the iteration:
各項目の整数インデックスとその値が必要な場合は、代わりに enumerated()
メソッドを使用して配列を反復処理します。 配列内の項目ごとに、enumerated()
メソッドは整数と項目で構成されるタプルを返します。 整数は 0
から始まり、項目ごとに 1 ずつカウントアップします。 配列全体を列挙する場合、これらの整数は項目のインデックスと一致します。 反復の一部として、タプルを一時的な定数または変数に分解できます。
for (index, value) in shoppingList.enumerated() {
print("Item \(index + 1): \(value)")
}
// Item 1: Six eggs
// Item 2: Milk
// Item 3: Flour
// Item 4: Baking Powder
// Item 5: Bananas
For more about the for
–in
loop, see For-In Loops.
for-in
ループの詳細については、「For-In ループ」を参照してください。
Sets
セット
A set stores distinct values of the same type in a collection with no defined ordering. You can use a set instead of an array when the order of items isn’t important, or when you need to ensure that an item only appears once.
セットは、順序が定義されていないコレクション内の同じ型の個別の値を保存します。 項目の順序が重要ではない場合、または項目が 1 回だけ表示されるようにする必要がある場合は、配列の代わりにセットを使用できます。
Note
注釈
Swift’s Set
type is bridged to Foundation’s NSSet
class.
Swift の Set
型は Foundation の NSSet
クラスにブリッジされます。
For more information about using Set
with Foundation and Cocoa, see Bridging Between Set and NSSet(Link:developer.apple.com).
Foundation および Cocoa で Set
を使用する方法の詳細については、「Set と NSSet の間のブリッジング」(Link:developer.apple.com)(英語)を参照してください。
Hash Values for Set Types
セット型のハッシュ値
A type must be hashable in order to be stored in a set — that is, the type must provide a way to compute a hash value for itself. A hash value is an Int
value that’s the same for all objects that compare equally, such that if a == b
, the hash value of a
is equal to the hash value of b
.
型はセットに保存されるためにハッシュ可能である必要があります。つまり、型はそれ自体のhash valueを計算する方法を提供する必要があります。 ハッシュ値は、同等に比較されるすべてのオブジェクトで同じ Int
値です。たとえば、a == b
の場合、a
のハッシュ値は b
のハッシュ値と等しくなります。
All of Swift’s basic types (such as String
, Int
, Double
, and Bool
) are hashable by default, and can be used as set value types or dictionary key types. Enumeration case values without associated values (as described in Enumerations) are also hashable by default.
Swift のすべての基本型(String
、Int
、Double
、Bool
など)はデフォルトでハッシュ可能であり、設定値型または辞書キー型として使用できます。 値が関連付けられていない列挙型のケース値(「列挙型」で説明)も、デフォルトでハッシュ可能です。
Note
注釈
You can use your own custom types as set value types or dictionary key types by making them conform to the Hashable
protocol from the Swift standard library. For information about implementing the required hash(into:)
method, see Hashable
(Link:developer.apple.com). For information about conforming to protocols, see Protocols.
独自のカスタム 型を Swift 標準ライブラリの Hashable
プロトコルに準拠させることで、設定値型または辞書キー型として使用できます。 必要な hash(into:)
メソッドの実装については、「Hashable
(Link:developer.apple.com)(英語)」を参照してください。 プロトコルへの準拠については、「Protocols」をご覧ください。
Set Type Syntax
セット型の構文
The type of a Swift set is written as Set<Element>
, where Element
is the type that the set is allowed to store. Unlike arrays, sets don’t have an equivalent shorthand form.
Swift セットの型は Set<Element>
として記述されます。ここで、Element
はセットが保存できる型です。 配列とは異なり、セットには同等の省略形がありません。
Creating and Initializing an Empty Set
空のセットの作成と初期化
You can create an empty set of a certain type using initializer syntax:
イニシャライザ構文を使用して、特定の型の空のセットを作成できます。
var letters = Set<Character>()
print("letters is of type Set<Character> with \(letters.count) items.")
// Prints "letters is of type Set<Character> with 0 items."
Note
注釈
The type of the letters
variable is inferred to be Set<Character>
, from the type of the initializer.
letters
変数の型は、初期化子の型から Set<Character>
であると推測されます。
Alternatively, if the context already provides type information, such as a function argument or an already typed variable or constant, you can create an empty set with an empty array literal:
あるいは、コンテキストが関数の引数やすでに型指定された変数や定数などの型情報をすでに提供している場合は、空の配列リテラルで空のセットを作成できます。
letters.insert("a")
// letters now contains 1 value of type Character
letters = []
// letters is now an empty set, but is still of type Set<Character>
Creating a Set with an Array Literal
配列リテラルを使用したセットの作成
You can also initialize a set with an array literal, as a shorthand way to write one or more values as a set collection.
1 つ以上の値をセット コレクションとして書き込む簡単な方法として、配列リテラルを使用してセットを初期化することもできます。
The example below creates a set called favoriteGenres
to store String
values:
以下の例では、String
値を保存するために、favoriteGenres
というセットを作成します。
var favoriteGenres: Set<String> = ["Rock", "Classical", "Hip hop"]
// favoriteGenres has been initialized with three initial items
The favoriteGenres
variable is declared as “a set of String
values”, written as Set<String>
. Because this particular set has specified a value type of String
, it’s only allowed to store String
values. Here, the favoriteGenres
set is initialized with three String
values ("Rock"
, "Classical"
, and "Hip hop"
), written within an array literal.
favouriteGenres
変数は「String
値のセット」として宣言され、Set<String>
として記述されます。 この特定のセットでは値の型としてString
が指定されているため、String
値のみを保存できます。 ここで、favoriteGenres
セットは、配列リテラル内に記述された 3 つの String 値 (("Rock"
、"Classical"
、"Hip hop"
) で初期化されます。
Note
注釈
The favoriteGenres
set is declared as a variable (with the var
introducer) and not a constant (with the let
introducer) because items are added and removed in the examples below.
以下の例では項目が追加および削除されるため、favoriteGenres
セットは定数 (let
イントロデューサーを使用) ではなく変数 (var
イントロデューサーを使用) として宣言されています。
A set type can’t be inferred from an array literal alone, so the type Set
must be explicitly declared. However, because of Swift’s type inference, you don’t have to write the type of the set’s elements if you’re initializing it with an array literal that contains values of just one type. The initialization of favoriteGenres
could have been written in a shorter form instead:
セット型は配列リテラルだけからは推論できないため、型 Set を明示的に宣言する必要があります。 ただし、Swift の型推論により、1 つの型の値のみを含む配列リテラルでセットを初期化する場合は、セットの要素の型を記述する必要はありません。 favouriteGenres
の初期化は、代わりに短い形式で記述することもできます。
var favoriteGenres: Set = ["Rock", "Classical", "Hip hop"]
Because all values in the array literal are of the same type, Swift can infer that Set<String>
is the correct type to use for the favoriteGenres
variable.
配列リテラル内の値はすべて同じ型であるため、Swift は Set<String>
が favouriteGenres
変数に使用する正しい型であると推測できます。
Accessing and Modifying a Set
セットへのアクセスと変更
You access and modify a set through its methods and properties.
セットのメソッドとプロパティを通じてセットにアクセスし、変更します。
To find out the number of items in a set, check its read-only count
property:
セット内のアイテムの数を確認するには、その読み取り専用の count
プロパティを確認します。
print("I have \(favoriteGenres.count) favorite music genres.")
// Prints "I have 3 favorite music genres."
Use the Boolean isEmpty
property as a shortcut for checking whether the count
property is equal to 0
:
count
プロパティが 0
に等しいかどうかを確認するためのショートカットとして、Boolean isEmpty
プロパティを使用します。
if favoriteGenres.isEmpty {
print("As far as music goes, I'm not picky.")
} else {
print("I have particular music preferences.")
}
// Prints "I have particular music preferences."
You can add a new item into a set by calling the set’s insert(_:)
method:
セットの insert(_:)
メソッドを呼び出すことで、セットに新しいアイテムを追加できます。
favoriteGenres.insert("Jazz")
// favoriteGenres now contains 4 items
You can remove an item from a set by calling the set’s remove(_:)
method, which removes the item if it’s a member of the set, and returns the removed value, or returns nil
if the set didn’t contain it. Alternatively, all items in a set can be removed with its removeAll()
method.
セットからアイテムを削除するには、セットのremove(_:)
メソッドを呼び出します。このメソッドは、アイテムがセットのメンバーである場合はアイテムを削除し、削除された値を返します。セットにアイテムが含まれていない場合はnil
を返します。 あるいは、セット内のすべてのアイテムは、removeAll()
メソッドを使用して削除できます。
if let removedGenre = favoriteGenres.remove("Rock") {
print("\(removedGenre)? I'm over it.")
} else {
print("I never much cared for that.")
}
// Prints "Rock? I'm over it."
To check whether a set contains a particular item, use the contains(_:)
method.
セットに特定のアイテムが含まれているかどうかを確認するには、contains(_:)
メソッドを使用します。
if favoriteGenres.contains("Funk") {
print("I get up on the good foot.")
} else {
print("It's too funky in here.")
}
// Prints "It's too funky in here."
Iterating Over a Set
セットの反復
You can iterate over the values in a set with a for
–in
loop.
for-in
ループを使用して、セット内の値を反復処理できます。
for genre in favoriteGenres {
print("\(genre)")
}
// Classical
// Jazz
// Hip hop
For more about the for
–in
loop, see For-In Loops.
for-in
ループの詳細については、「For-In ループ」を参照してください。
Swift’s Set
type doesn’t have a defined ordering. To iterate over the values of a set in a specific order, use the sorted()
method, which returns the set’s elements as an array sorted using the <
operator.
Swift の Set
型には定義された順序がありません。 セットの値を特定の順序で反復処理するには、sorted()
メソッドを使用します。このメソッドは、<
演算子を使用して並べ替えられた配列としてセットの要素を返します。
for genre in favoriteGenres.sorted() {
print("\(genre)")
}
// Classical
// Hip hop
// Jazz
Performing Set Operations
集合演算の実行
You can efficiently perform fundamental set operations, such as combining two sets together, determining which values two sets have in common, or determining whether two sets contain all, some, or none of the same values.
2 つのセットを組み合わせたり、2 つのセットに共通する値を特定したり、2 つのセットに同じ値がすべて含まれているか、一部が含まれているか、まったく含まれていないかを判断するなど、基本的なセット演算を効率的に実行できます。
Fundamental Set Operations
基本的な集合演算
The illustration below depicts two sets — a
and b
— with the results of various set operations represented by the shaded regions.
以下の図は、2 つのセット a と b を示しており、さまざまなセット演算の結果が影付きの領域で表されています。

- Use the
intersection(_:)
method to create a new set with only the values common to both sets. intersection(_:)
メソッドを使用して、両方のセットに共通の値のみを含む新しいセットを作成します。- Use the
symmetricDifference(_:)
method to create a new set with values in either set, but not both. symmetricDifference(_:)
メソッドを使用して、両方ではなくいずれかのセットの値を含む新しいセットを作成します。- Use the
union(_:)
method to create a new set with all of the values in both sets. Union(_:)
メソッドを使用して、両方のセットのすべての値を含む新しいセットを作成します。- Use the
subtracting(_:)
method to create a new set with values not in the specified set. - 指定されたセットにない値を含む新しいセットを作成するには、
subtracting(_:)
メソッドを使用します。
let oddDigits: Set = [1, 3, 5, 7, 9]
let evenDigits: Set = [0, 2, 4, 6, 8]
let singleDigitPrimeNumbers: Set = [2, 3, 5, 7]
oddDigits.union(evenDigits).sorted()
// [0, 1, 2, 3, 4, 5, 6, 7, 8, 9]
oddDigits.intersection(evenDigits).sorted()
// []
oddDigits.subtracting(singleDigitPrimeNumbers).sorted()
// [1, 9]
oddDigits.symmetricDifference(singleDigitPrimeNumbers).sorted()
// [1, 2, 9]
Set Membership and Equality
メンバーシップと平等性を設定する
The illustration below depicts three sets — a
, b
and c
— with overlapping regions representing elements shared among sets. Set a
is a superset of set b
, because a
contains all elements in b
. Conversely, set b
is a subset of set a
, because all elements in b
are also contained by a
. Set b
and set c
are disjoint with one another, because they share no elements in common.
下の図は、3 つのセット (a、b、c) を示しており、重なり合う領域はセット間で共有される要素を表しています。 a には b のすべての要素が含まれているため、セット a はセット b のスーパーセットです。 逆に、b のすべての要素は a にも含まれるため、セット b はセット a のサブセットです。 セット b とセット c は共通の要素がないため、互いに素です。

- Use the “is equal” operator (
==
) to determine whether two sets contain all of the same values. - 「等しい」演算子 (
==
) を使用して、2 つのセットにすべて同じ値が含まれているかどうかを判断します。 - Use the
isSubset(of:)
method to determine whether all of the values of a set are contained in the specified set. isSubset(of:)
メソッドを使用して、セットのすべての値が指定されたセットに含まれているかどうかを確認します。- Use the
isSuperset(of:)
method to determine whether a set contains all of the values in a specified set. isSuperset(of:)
メソッドを使用して、指定されたセット内のすべての値がセットに含まれているかどうかを確認します。- Use the
isStrictSubset(of:)
orisStrictSuperset(of:)
methods to determine whether a set is a subset or superset, but not equal to, a specified set. isStrictSubset(of:)
メソッドまたはisStrictSuperset(of:)
メソッドを使用して、セットがサブセットであるかスーパーセットであるが、指定されたセットと等しくないかを判断します。- Use the
isDisjoint(with:)
method to determine whether two sets have no values in common. isDisjoint(with:)
メソッドを使用して、2 つのセットに共通の値がないかどうかを判断します。
let houseAnimals: Set = ["🐶", "🐱"]
let farmAnimals: Set = ["🐮", "🐔", "🐑", "🐶", "🐱"]
let cityAnimals: Set = ["🐦", "🐭"]
houseAnimals.isSubset(of: farmAnimals)
// true
farmAnimals.isSuperset(of: houseAnimals)
// true
farmAnimals.isDisjoint(with: cityAnimals)
// true
Dictionaries
辞書
A dictionary stores associations between keys of the same type and values of the same type in a collection with no defined ordering. Each value is associated with a unique key, which acts as an identifier for that value within the dictionary. Unlike items in an array, items in a dictionary don’t have a specified order. You use a dictionary when you need to look up values based on their identifier, in much the same way that a real-world dictionary is used to look up the definition for a particular word.
dictionaryは、順序が定義されていないコレクション内の同じ型のキーと同じ型の値の間の関連付けを保存します。 各値は一意のキーに関連付けられており、辞書内でその値の識別子として機能します。 配列内の項目とは異なり、辞書内の項目には指定された順序がありません。 特定の単語の定義を調べるために実際の辞書を使用するのと同じように、識別子に基づいて値を検索する必要がある場合に辞書を使用します。
Note
注釈
Swift’s Dictionary
type is bridged to Foundation’s NSDictionary
class.
Swift の Dictionary
型は Foundation の NSDictionary
クラスにブリッジされます。
For more information about using Dictionary
with Foundation and Cocoa, see Bridging Between Dictionary and NSDictionary(Link:developer.apple.com).
Foundation および Cocoa での Dictionary の使用の詳細については、「Dictionary と NSDictionary の間のブリッジング(Link:developer.apple.com)(英語)」を参照してください。
Dictionary Type Shorthand Syntax
辞書型の短縮構文
The type of a Swift dictionary is written in full as Dictionary<Key, Value>
, where Key
is the type of value that can be used as a dictionary key, and Value
is the type of value that the dictionary stores for those keys.
Swift 辞書の型は、完全に Dictionary として記述されます。ここで、Key は辞書キーとして使用できる値の型、Value はそれらのキーに対して辞書が保存する値の型です。
Note
注釈
A dictionary Key
type must conform to the Hashable
protocol, like a set’s value type.
辞書のキー 型は、セットの値の型と同様に、Hashable
protocolに準拠する必要があります。
You can also write the type of a dictionary in shorthand form as [Key: Value]
. Although the two forms are functionally identical, the shorthand form is preferred and is used throughout this guide when referring to the type of a dictionary.
辞書の種類を [Key: Value]
という省略形で記述することもできます。 2 つの形式は機能的には同じですが、辞書の種類を参照する場合は省略形式が好まれ、このガイド全体で使用されます。
Creating an Empty Dictionary
空の辞書の作成
As with arrays, you can create an empty Dictionary
of a certain type by using initializer syntax:
配列と同様に、初期化子構文を使用して、特定の型の空の辞書を作成できます。
var namesOfIntegers: [Int: String] = [:]
// namesOfIntegers is an empty [Int: String] dictionary
This example creates an empty dictionary of type [Int: String]
to store human-readable names of integer values. Its keys are of type Int
, and its values are of type String
.
この例では、[Int: String] 型の空の辞書を作成し、人間が判読できる整数値の名前を保存します。 そのキーの型は Int で、値の型は String です。
If the context already provides type information, you can create an empty dictionary with an empty dictionary literal, which is written as [:]
(a colon inside a pair of square brackets):
コンテキストがすでに型情報を提供している場合は、[:]
(角かっこ内のコロン)として記述される空の辞書リテラルを含む空の辞書を作成できます。
namesOfIntegers[16] = "sixteen"
// namesOfIntegers now contains 1 key-value pair
namesOfIntegers = [:]
// namesOfIntegers is once again an empty dictionary of type [Int: String]
Creating a Dictionary with a Dictionary Literal
辞書リテラルを使用した辞書の作成
You can also initialize a dictionary with a dictionary literal, which has a similar syntax to the array literal seen earlier. A dictionary literal is a shorthand way to write one or more key-value pairs as a Dictionary
collection.
辞書リテラルを使用して辞書を初期化することもできます。これは、前に示した配列リテラルと同様の構文を持ちます。 辞書リテラルは、1 つ以上のキーと値のペアを辞書コレクションとして記述する簡単な方法です。
A key-value pair is a combination of a key and a value. In a dictionary literal, the key and value in each key-value pair are separated by a colon. The key-value pairs are written as a list, separated by commas, surrounded by a pair of square brackets:
Key-Value ペアは、キーと値の組み合わせです。 辞書リテラルでは、各キーと値のペアのキーと値はコロンで区切られます。 キーと値のペアは、カンマで区切られ、角かっこで囲まれたリストとして記述されます。
[<#key 1#>: <#value 1#>, <#key 2#>: <#value 2#>, <#key 3#>: <#value 3#>]
The example below creates a dictionary to store the names of international airports. In this dictionary, the keys are three-letter International Air Transport Association codes, and the values are airport names:
以下の例では、国際空港の名前を格納する辞書を作成します。 このディクショナリでは、キーは 3 文字の国際航空運送協会コード、値は空港名です。
var airports: [String: String] = ["YYZ": "Toronto Pearson", "DUB": "Dublin"]
The airports
dictionary is declared as having a type of [String: String]
, which means “a Dictionary
whose keys are of type String
, and whose values are also of type String
”.
Airports
辞書は、[String: String]
型を持つものとして宣言されています。これは、「キーが String
型で、値も String
型であるDictionary
」を意味します。
Note
注釈
The airports
dictionary is declared as a variable (with the var
introducer), and not a constant (with the let
introducer), because more airports are added to the dictionary in the examples below.
以下の例ではさらに多くの空港が辞書に追加されているため、airports 辞書は定数 (let イントロデューサを使用) ではなく変数 (var イントロデューサを使用) として宣言されています。
The airports
dictionary is initialized with a dictionary literal containing two key-value pairs. The first pair has a key of "YYZ"
and a value of "Toronto Pearson"
. The second pair has a key of "DUB"
and a value of "Dublin"
.
Airports
辞書は、2 つのキーと値のペアを含む辞書リテラルで初期化されます。 最初のペアのキーは"YYZ"
、値は"Toronto Pearson"
です。 2 番目のペアのキーは"DUB"
、値は"Dublin"
です。
This dictionary literal contains two String: String
pairs. This key-value type matches the type of the airports
variable declaration (a dictionary with only String
keys, and only String
values), and so the assignment of the dictionary literal is permitted as a way to initialize the airports
dictionary with two initial items.
この辞書リテラルには、2 つの String: String
ペアが含まれています。 この Key-Value 型は、airports
変数宣言の型(String
キーのみとString
値のみを含む辞書)と一致するため、2 つの初期項目で空港辞書を初期化する方法として、辞書リテラルの割り当てが許可されます。
As with arrays, you don’t have to write the type of the dictionary if you’re initializing it with a dictionary literal whose keys and values have consistent types. The initialization of airports
could have been written in a shorter form instead:
配列の場合と同様、キーと値の型が一貫している辞書リテラルを使用して辞書を初期化する場合は、辞書の型を記述する必要はありません。 空港の初期化は、代わりに短い形式で記述することもできます。
var airports = ["YYZ": "Toronto Pearson", "DUB": "Dublin"]
Because all keys in the literal are of the same type as each other, and likewise all values are of the same type as each other, Swift can infer that [String: String]
is the correct type to use for the airports
dictionary.
リテラル内のすべてのキーは互いに同じ型であり、同様にすべての値も互いに同じ型であるため、Swift は [String: String]
がairports
辞書に使用する正しい型であると推測できます。
Accessing and Modifying a Dictionary
辞書へのアクセスと変更
You access and modify a dictionary through its methods and properties, or by using subscript syntax.
ディクショナリにアクセスして変更するには、そのメソッドとプロパティを使用するか、添え字構文を使用します。
As with an array, you find out the number of items in a Dictionary
by checking its read-only count
property:
配列と同様に、辞書内の項目数を確認するには、読み取り専用の count
プロパティを確認します。
print("The airports dictionary contains \(airports.count) items.")
// Prints "The airports dictionary contains 2 items."
Use the Boolean isEmpty
property as a shortcut for checking whether the count
property is equal to 0
:
count
プロパティが 0
に等しいかどうかを確認するためのショートカットとして、Boolean isEmpty
プロパティを使用します。
if airports.isEmpty {
print("The airports dictionary is empty.")
} else {
print("The airports dictionary isn't empty.")
}
// Prints "The airports dictionary isn't empty."
You can add a new item to a dictionary with subscript syntax. Use a new key of the appropriate type as the subscript index, and assign a new value of the appropriate type:
添字構文を使用して新しい項目を辞書に追加できます。 適切な型の新しいキーを添え字インデックスとして使用し、適切な型の新しい値を割り当てます。
airports["LHR"] = "London"
// the airports dictionary now contains 3 items
You can also use subscript syntax to change the value associated with a particular key:
添え字構文を使用して、特定のキーに関連付けられた値を変更することもできます。
airports["LHR"] = "London Heathrow"
// the value for "LHR" has been changed to "London Heathrow"
As an alternative to subscripting, use a dictionary’s updateValue(_:forKey:)
method to set or update the value for a particular key. Like the subscript examples above, the updateValue(_:forKey:)
method sets a value for a key if none exists, or updates the value if that key already exists. Unlike a subscript, however, the updateValue(_:forKey:)
method returns the old value after performing an update. This enables you to check whether or not an update took place.
添え字の代わりに、辞書の updateValue(:forKey:)
メソッドを使用して、特定のキーの値を設定または更新します。 上記の添え字の例と同様、updateValue(:forKey:)
メソッドは、キーが存在しない場合は値を設定し、キーがすでに存在する場合は値を更新します。 ただし、添え字とは異なり、updateValue(_:forKey:)
メソッドは更新を実行した後に古い値を返します。 これにより、アップデートが行われたかどうかを確認できます。
The updateValue(_:forKey:)
method returns an optional value of the dictionary’s value type. For a dictionary that stores String
values, for example, the method returns a value of type String?
, or “optional String
”. This optional value contains the old value for that key if one existed before the update, or nil
if no value existed:
updateValue(_:forKey:)
メソッドは、辞書の値型のオプショナル値を返します。 たとえば、String
値を格納する辞書の場合、メソッドはString?
、または“optional String
”型の値を返します。 このオプショナル値には、更新前にキーが存在していた場合はそのキーの古い値が含まれ、値が存在しなかった場合は nil が含まれます。
if let oldValue = airports.updateValue("Dublin Airport", forKey: "DUB") {
print("The old value for DUB was \(oldValue).")
}
// Prints "The old value for DUB was Dublin."
You can also use subscript syntax to retrieve a value from the dictionary for a particular key. Because it’s possible to request a key for which no value exists, a dictionary’s subscript returns an optional value of the dictionary’s value type. If the dictionary contains a value for the requested key, the subscript returns an optional value containing the existing value for that key. Otherwise, the subscript returns nil
:
添え字構文を使用して、辞書から特定のキーの値を取得することもできます。 値が存在しないキーをリクエストすることもできるため、ディクショナリの添字はディクショナリの値型のオプショナル値を返します。 ディクショナリに要求されたキーの値が含まれている場合、添え字はそのキーの既存の値を含むオプションの値を返します。 それ以外の場合、添え字は nil
を返します。
if let airportName = airports["DUB"] {
print("The name of the airport is \(airportName).")
} else {
print("That airport isn't in the airports dictionary.")
}
// Prints "The name of the airport is Dublin Airport."
You can use subscript syntax to remove a key-value pair from a dictionary by assigning a value of nil
for that key:
添え字構文を使用して、キーに値 nil
を割り当てることで、キーと値のペアを辞書から削除できます。
airports["APL"] = "Apple International"
// "Apple International" isn't the real airport for APL, so delete it
airports["APL"] = nil
// APL has now been removed from the dictionary
Alternatively, remove a key-value pair from a dictionary with the removeValue(forKey:)
method. This method removes the key-value pair if it exists and returns the removed value, or returns nil
if no value existed:
または、removeValue(forKey:)
メソッドを使用して辞書から Key-Value ペアを削除します。 このメソッドは、キーと値のペアが存在する場合はそれを削除し、削除された値を返します。値が存在しない場合は nil
を返します。
if let removedValue = airports.removeValue(forKey: "DUB") {
print("The removed airport's name is \(removedValue).")
} else {
print("The airports dictionary doesn't contain a value for DUB.")
}
// Prints "The removed airport's name is Dublin Airport."
Iterating Over a Dictionary
辞書の反復処理
You can iterate over the key-value pairs in a dictionary with a for
–in
loop. Each item in the dictionary is returned as a (key, value)
tuple, and you can decompose the tuple’s members into temporary constants or variables as part of the iteration:
for-in
ループを使用して、辞書内の Key-Value ペアを反復処理できます。 辞書内の各項目は(key, value)
タプルとして返され、反復の一部としてタプルのメンバーを一時的な定数または変数に分解できます。
for (airportCode, airportName) in airports {
print("\(airportCode): \(airportName)")
}
// LHR: London Heathrow
// YYZ: Toronto Pearson
For more about the for
–in
loop, see For-In Loops.
for-in
ループの詳細については、「For-In ループ」を参照してください。
You can also retrieve an iterable collection of a dictionary’s keys or values by accessing its keys
and values
properties:
辞書のキーと値のプロパティにアクセスして、辞書のキーまたは値の反復可能なコレクションを取得することもできます。
for airportCode in airports.keys {
print("Airport code: \(airportCode)")
}
// Airport code: LHR
// Airport code: YYZ
for airportName in airports.values {
print("Airport name: \(airportName)")
}
// Airport name: London Heathrow
// Airport name: Toronto Pearson
If you need to use a dictionary’s keys or values with an API that takes an Array
instance, initialize a new array with the keys
or values
property:
Array
インスタンスを受け取る API で辞書のキーまたは値を使用する必要がある場合は、 keys
またはvalues
のプロパティを使用して新しい配列を初期化します。
let airportCodes = [String](airports.keys)
// airportCodes is ["LHR", "YYZ"]
let airportNames = [String](airports.values)
// airportNames is ["London Heathrow", "Toronto Pearson"]
Swift’s Dictionary
type doesn’t have a defined ordering. To iterate over the keys or values of a dictionary in a specific order, use the sorted()
method on its keys
or values
property.
Swift の Dictionary
型には、定義された順序がありません。 辞書の keys
またはvalues
を特定の順序で反復処理するには、そのキーまたは値のプロパティでsorted()
メソッドを使用します。